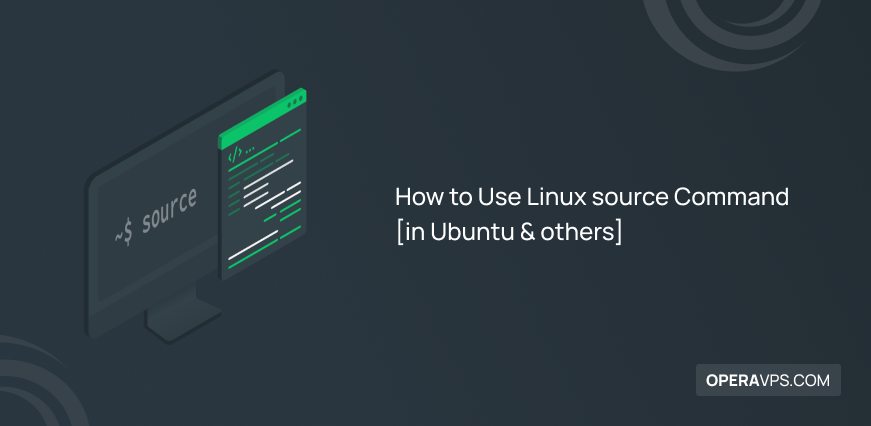
How to Use Linux source Command
The Linux source command is used to execute commands from a specified file within the current shell environment. This is equivalent to running the commands directly in the terminal.
It’s often used to load configuration files, environment variables, or aliases into the current shell session.
Here is the basic syntax of source command in Linux Ubuntu and others:
source filename [options]
- filename: The path to the file containing the commands to be executed.
- options: Any options you pass in while running the file are converted to positional options.
Prerequisites to Use Linux source Command
Provide the options below to let this tutorial work correctly and move on.
- A Linux VPS running a compatible Linux distribution (Ubuntu, Debian, CentOS).
- A non-root user with
sudo
privileges. - Access to Terminal/Command line.
Practical Examples of Linux source Command
Let’s go through the examples of this tutorial to use the source command effectively, and enhance your Linux shell experience and streamline your workflows.
1. Using source command to Read Configuration Files
When you execute the source
command in Linux, the shell reads the contents of the configuration file line by line and executes the commands within it.
The source
command requires the full path to the configuration file.
- Syntax:
source configuration_file
Note: You need to replace configuration_file
with the actual path to the configuration file you want to read.
- Example:
source .bashrc
In this example, .bashrc
is a relative path, assuming it’s in the current user’s home directory. There are also other configuration files for different shells (e.g., .zshrc
for Zsh) and applications (e.g., .npmrc
for npm).
This command reads the .bashrc file, which is a common configuration file for the Bash shell. It’s often located in the user’s home directory.
Note: Configuration files often contain commands to set environment variables. For example, .bashrc
might set variables like PATH
, PS1
, or EDITOR
.
2. Using Linux source command to Centralize Reusable Functions
When you need to use a function frequently to check if the current user has root privileges, you can use source functions.
Here’s how to create a reusable function and integrate it into your scripts using source command in Linux (Ubuntu, Debian, CentOS,…):
- Create a Function File (check_root.sh)
#!/bin/bash
function check_root() {
if [[ $EUID -ne 0 ]]; then
echo "This script requires root privileges."
exit 1
fi
}
Notes:
- Save this file in a convenient location (e.g.,
/usr/local/bin/check_root.sh
or your home directory). - Make sure the file has execute permissions (use
chmod +x check_root.sh
).
- Source the Function in Your Script (example_script.sh)
#!/bin/bash
source check_root.sh # Load the check_root function
# Rest of your script's code
echo "This is the root user" # This will only be printed if the user is root
Remember to replace # Rest of your script's code
with your desired actions.
- Run the Script
# As a non-root user (will fail)
bash example_script.sh
# As a root user (will print "This is the root user")
sudo bash example_script.sh
3. Using source command to Refresh Environment Variables
When you source a configuration file, it’s like re-executing the commands defined within it, effectively refreshing the shell’s environment.
Let’s check the examples of common use cases for Linux source command to refresh the Shell Environment.
- Updating Environment Variables:
Example:
# .bashrc
export PATH="$HOME/.local/bin:$PATH"
To update the PATH
variable after installing a new tool, you can source .bashrc
:
source .bashrc
- Reloading Aliases and Functions:
Example:
# .bashrc
alias ll='ls -al'
If you modify the ll
alias, sourcing .bashrc
will make the new definition available:
source .bashrc
- Reloading Shell Prompts:
Example:
# .bashrc
PS1='\u@\h:\w\$ '
To apply a new prompt, source .bashrc
:
- Loading Custom Configurations:
Create a custom configuration file (e.g., my_config.sh
) with your desired settings:
# my_config.sh
export MY_VAR="value"
alias my_command="echo Hello"
Source the file to apply the settings:
source my_config.sh
4. Using source command in Linux to Load Environment Variables Dynamically
To create a file named environment.sh
with the following content, run:
#!/bin/bash
export MY_VARIABLE="Hello, world!"
export ANOTHER_VARIABLE=42
- Example:
1. Source the file:
source environment.sh
2. Access the variables:
echo $MY_VARIABLE
echo $ANOTHER_VARIABLE
5. Using source command to Execute Shell Scripts
The source command allows you to directly include the functionality of your script within the current shell session.
Variables and functions defined in the script can be accessed and used within your current shell session, making them reusable.
- Syntax:
source script_name.sh
Notes:
- Replace
script_name.sh
with the actual filename of your shell script. - The script file must have execute permissions (
chmod +x script_name.sh
). - Changes made within the sourced script might not persist after the current shell session ends.
- Example:
script_name.sh:
#!/bin/bash
# Function to calculate the area of a rectangle
function calculate_area {
length=$1
width=$2
area=$(( length * width ))
echo "The area of the rectangle is $area square units."
}
# Example usage
calculate_area 5 3
Running the Script with source
:
source script_name.sh
calculate_area 10 4
- The
calculate_area
function is defined inscript_name.sh
. - When you source the script, the function becomes accessible in your current shell.
- You can then call
calculate_area
with arguments to calculate and print the area. - Be cautious when sourcing scripts from untrusted sources, as they might contain malicious code.
6. Using source command to Switch between Shells
You can use source command in Linux to switch between different shell environments for specific tasks or projects.
This involves setting different environment variables, aliases, and configurations. The source
command can be a convenient tool for this.
- Syntax:
source environment_config.sh
Replace environment_config.sh
with the actual filename of your configuration script.
- Example:
1. Create Configuration Scripts:
development.sh:
export PATH="$HOME/.local/bin:$PATH"
alias dev_command="echo Development mode"
production.sh:
export PATH="$HOME/projects/my_project/bin:$PATH"
alias prod_command="echo Production mode"
2. Switch Between Environments:
# Switch to development environment
source development.sh
# Switch to production environment
source production.sh
7. Using Linux source command to Change Shell Prompts
Consider creating a file named prompt.sh
with the following content:
#!/bin/bash
PS1="\u@\h:\w\$ "
- Example:
1. Source the file:
source prompt.sh
2. Observe the new prompt: Open a new terminal or re-open the current one to see the changed prompt.
That’s it! By mastering the source
command, you can create a personalized and efficient shell experience.
What is Linux source command and How does it work?
The source
command in Linux is a powerful tool that allows you to execute commands from a specified file within the current shell environment.
It’s akin to copying and pasting the contents of the file directly into your terminal, but with the added benefit of maintaining the context of the current shell session.
When you use source
, the commands within the specified file are executed as if they were typed directly into the terminal, ensuring that any changes made take effect immediately in your current shell session.
What does source command do in Linux?
Loads Configuration Files: source is commonly used to load configuration files like .bashrc
or .zshrc
, which define environment variables, aliases, and other shell settings.
Executes Scripts: You can use source to run shell scripts directly within your current shell session, making their functions and variables available immediately.
Updates Environment Variables: By sourcing a configuration file, you can dynamically update environment variables without having to restart your shell.
Reloads Aliases and Functions: If you modify aliases or functions in your configuration files, sourcing them will make the changes effective in your current shell session.
Customizes Shell Behavior: source allows you to tailor your shell environment to your preferences by loading custom configuration files.
What are the differences between source and bash?
Feature | source | bash |
---|---|---|
Purpose | Executes commands from a file within the current shell environment | Creates a new subshell to execute commands |
Context | Modifies the current shell's environment variables, aliases, and functions | Executes commands in a separate environment, preserving the original shell's state |
Usage | source filename | bash filename |
Scope | Changes made by source affect the current shell | Changes made within the bash subshell are isolated |
Example | Load configuration file: source .bashrc | Execute a script: bash my_script.sh |
What is the difference between source and ./script.sh?
Both execute scripts, but source
runs the script within the current shell environment, while ./script.sh
creates a new subshell.
Can I use source to run scripts that require root privileges?
Yes, but you’ll need to use sudo to run the source
command with root privileges.
For example: sudo source my_script.sh
How to Troubleshoot Not Executing my Script after Using source?
- Check if the script has executable permissions (
use chmod +x script.sh
). - Ensure there are no syntax errors in the script.
- Verify that the script is in the correct path.
Conclusion
The source
command is a versatile tool in Linux that empowers you to execute commands from a file within your current shell session.
The explained source command examples in this guide help you know how to use source
command in Linux Ubuntu and others.
Now you can Load configuration files, set environment variables, define aliases, and customize shell behavior using the Linux source
command.
Tools like Ansible or Puppet can help manage and automate the use of source
for configuration management.